Creating patterned Polymers
A Polymer can be constructed as a given combination of monomer(-s). Sometimes, there is the need to create combination of these units, which can be achieved in PySoftK by employing the pysoftk.topologies.diblock
function. First, we need to import the initial molecules (stored in a Python list) using RDKit, as shown in the following snippet:
import rdkit
from rdkit import Chem
from rdkit.Chem import AllChem
mols=[Chem.MolFromSmiles('c1(ccc(cc1)Br)Br'),
Chem.MolFromSmiles('c1cc(oc1Br)Br'),
Chem.MolFromSmiles('c1cc(sc1Br)Br')]
Then, we can create a patterned topology, by defining a string that contains a pattern and then parsed to the corresponding function as shown below:
from pysoftk.topologies.diblock import *
string="ABC"
patt=Pt(string, mols, "Br").pattern_block_poly()
In this case, we have used 3 monomers and they have been described an alphabetic order using the letters ABC, representing the order in which the moieties will be merged.
The new molecular unit (stored in the variable patt) can be used to form a patternd polymer. The structure can be printed in XYZ format by adding the following lines of code:
from pysoftk.format_printers.format_mol import *
Fmt(patt).xyz_print("patterned.xyz")
By using a common visualization program (such as VMD), the built structure ring.xyz can be displayed and the result as presented above
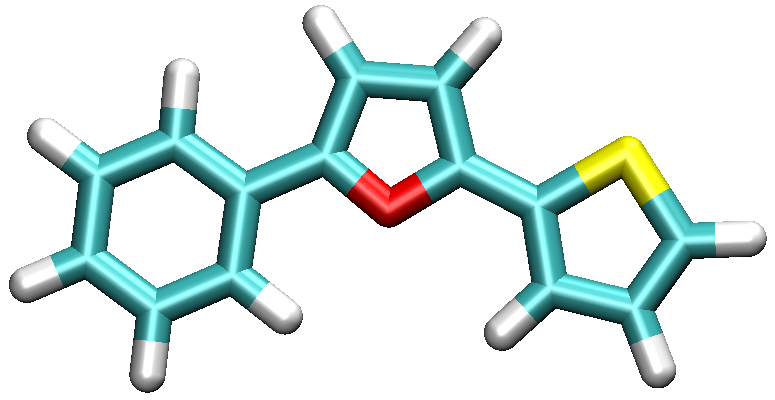
Figure Patterned polymer with 3 units (ABC).
Combinatorial creation of patterned Polymers
If the user is interested in creating libraries with different combinations for a given set of monomers, this can done by PySoftK with standard Python libraries such as itertools. As an example, we are going to create all possible permutations that can be generated a set of 3 different monomers. This displayed in the following snippet:
from itertools import permutations
alph1=permutations(["A", "B", "C"], 3)
string=[''.join(values) for idx, values in enumerate(alph1)]
for idx, values in enumerate(string):
patt=Pt(values, mols, "Br").pattern_block_poly()
Fmt(patt).xyz_print(values+".xyz")
This will create 6 different permutations ABC/ACB/BAC/BCA/CAB/CBA and all initial geometries are written in XYZ format. In the following section, we will present a functionality to organize files into folders developed in PySoftK.
![]() |
![]() |
![]() |
![]() |
![]() |
![]() |